The Java main() method serves as an entry point for an application. It is a block of code that is executed when the program is started. The main() method is required to start the execution of a program in Java and is a mandatory part of every Java application. Let us dive deeper into the details of the main() method in Java.
Syntax of main() method
In Java, the main() method is located within a class and serves as the starting point for the execution of a Java program. The main() method must be declared with the following signature:
public class HelloWorld {
public static void main(String[] args){
//Some logic here
}
}
This method is usually located inside a class that contains the entry point of the program. The class itself can be named whatever you want, but the main() method must always be called “main” and have the specified signature, otherwise the JVM will not be able to find the method and we will get the following error:
Error: Main method not found in class HelloWorld, please define the main method as:
public static void main(String[] args)
Run the program
To run a standalone Java program from the command line, you must first ensure that you have the Java Development Kit (JDK) installed on your computer. Once it is deployed, you can follow these steps to run your Java program:
- Compile the program: Use the javac command to compile your Java program. For example, if your program is called “HelloWorld.java”, you would run the following command: javac HelloWorld.java. This will generate a file called “HelloWorld.class”, which contains the bytecode for your program.
- Run the program: After compiling the program, you can use the java command to run it. If your program contains a main() method, you can run it by typing java HelloWorld in the command line. This will invoke the main() method and run the program.
For example, let’s say you have a simple Java program called “HelloWorld” with a main() method.
The project will be located in a base directory called «HelloWorld«. The «HelloWorld» directory will contain two subdirectories:
- The “src” subdirectory is intended to contain the source files (HelloWorld.java).
- The «bin» subdirectory that will contain the compiled files (HelloWorld.class).
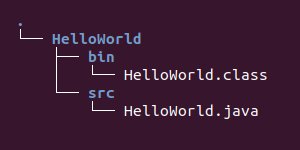
In the context of the “HelloWorld” project, the first step is to navigate to the project base directory using the command line. Once in the “HelloWorld” directory, the “HelloWorld.java” source code file should be placed in the “src” subdirectory and the compiled “HelloWorld.class” file should be placed in the “bin” subdirectory.
To compile the Java program from the command line, the javac command is used. While in the “HelloWorld” directory, the following command must be run to compile the “HelloWorld.java” file and place the compiled output in the “bin” directory:
javac -d bin src/HelloWorld.java
This command compiles the “HelloWorld.java” file and places the resulting “HelloWorld.class” file in the “bin” directory.
After successful compilation, the standalone Java program can be run using the java command. While in the “HelloWorld” directory, the following command must be run to run the compiled “HelloWorld.class” file from the “bin” directory:
java -cp bin HelloWorld
The -cp flag is used to specify the classpath and “HelloWorld” is the name of the class that contains the main() method. This command will invoke the main() method in the “HelloWorld” class and run the Java program.
How to pass arguments to the main() method
Passing arguments to the main() method provides a flexible means of interacting with a Java program, making it adaptable to a variety of use cases. Whether providing file paths, configuration parameters, or data input, understanding how to effectively handle command-line arguments enables you to create versatile, interactive applications.
Let us consider the following program:
public class HelloWorld {
public static void main(String[] args) {
if (args.length > 0){
System.out.println("-> Listado de argumentos ingresados:");
for (int i = 0; i < args.length; i++){
System.out.println("-> " + args[i]);
}
} else {
System.out.println("-> No se ingresaron argumentos.");
}
}
}
In this example, the main() method of the HelloWorld class accepts a String array as a parameter. This array contains the command line arguments passed when the program is run. The program checks to see if any arguments were passed, and if so, iterates through the array and prints each argument. If no arguments were passed, it simply prints a message indicating that.
When running this program from the command line, arguments can be passed after the program name, for example:
java -cp bin HelloWorld arg1 arg2 arg3
The output would then show:
-> Listado de argumentos ingresados:
-> arg1
-> arg2
-> arg3
Treating composite strings as a single parameter
You may encounter scenarios where a command line argument contains spaces and should be treated as a single parameter. For example, when passing a file path as an argument, such as “C:\Program Files\example.txt”, the entire path should be considered as a single parameter. To achieve this, you can enclose the composed string in double quotes during input and the Java program should handle it as a single argument during processing.
public class FileProcessor {
public static void main(String[] args) {
String filePath = args[0];
System.out.println("File path: " + filePath);
}
}
When running the Java program from the command line, the compound string can be passed as a single parameter enclosed in double quotes:
java -cp bin FileProcessor "C:\Program Files\example.txt"
Process a date
When a Java program requires date information as a command line argument, it becomes necessary to parse and process the supplied date. Suppose the program needs to process a date in the format “yyyy-MM-dd”. The main() method can parse the input string using the SimpleDateFormat class to ensure that it matches the required format and then process it within the program.
import java.text.SimpleDateFormat;
import java.util.Date;
public class DateProcessor {
public static void main(String[] args) {
if (args.length > 0) {
String inputDate = args[0];
try {
SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd");
Date date = dateFormat.parse(inputDate);
System.out.println("Fecha procesada: " + date);
} catch (java.text.ParseException e) {
System.err.println("Formato inválido. Por favor use yyyy-MM-dd.");
}
} else {
System.err.println("Por favor, ingrese una fecha como argumento.");
}
}
}
Conclusion
In this article we have reviewed the main features of the main() method in Java, and highlighted its relevance as an entry point for any standalone Java program.