In the world of programming, a “Hello World!” program serves as an introduction for people who are unfamiliar with a particular programming language, providing a simple example to demonstrate the basic syntax and functionality of the language. This article will guide you through the process of creating your first Java program, providing step-by-step instructions and explanations so you can understand the fundamental concepts of Java programming.
Prerequisites
Before you begin, there are some prerequisites to consider:
- Online Development Environment:
- The easiest way to learn Java and write your first Java program is to use an online development environment like Onecompiler. This eliminates the need to install the JDK, an IDE, or compile the code. You can simply open an online editor and start coding.
- Local Development Environment:
- If you prefer to work locally, you will need to install the Java Development Kit (JDK). In this case, you can install OpenJDK. However, using an online development environment eliminates the need for these installations.
- Text editor: To write the program you can use the text editor of your choice, although as you progress it is recommended that you install an Integrated Development Environment (IDE) such as Eclipse.
Write the program
The following is a program that when executed simply writes “Hello, World!” on the screen. The first step in making this program is to write the program listed below in our text editor and save it as “Main.java”.
public class Main {
public static void main(String[] args) {
System.out.println("Hola, Mundo!");
}
}
The Java program provided is a classic “Hello, World!” program that is often used as the first step for beginners learning a new programming language. This program simply prints the message “Hello, World!” to the console.
- The program starts with a class called “Main”, which is the main class of the program.
- Inside the “Main” class, there is a method called “main()”. This is a special method in Java that serves as an entry point to the program.
- Inside the main() method, there is a single line of code that uses the “System.out.println” command to print the message “Hello, World!” to the console.
- Class Definition:
- The line “public class Main” defines the class named “Main.” In Java, a class is a blueprint or template for creating objects. It contains methods and variables that define the behavior and state of objects created from it. In this program, the “Main” class serves as the starting point of the program.
- The main() method:
- The line “public static void main(String[] args)” declares the main() method. In Java, the “main()” method is the entry point for any standalone Java application. When the program is run, the Java Virtual Machine (JVM) starts executing the program from the “main()” method.
- Print text to console:
- The line “System.out.println(“Hello, World!”);” is responsible for printing the text “Hello, World!” to the console. In Java, “System.out” is an object that represents the standard output stream and “println” is a method used to print a line of text to the console. The text to be printed is enclosed in double quotes.
Compile and run
Once the program is written, we will open a terminal in the directory where the Main.java file is located.
To compile the program we use the javac compiler from the terminal as shown below:
javac Main.java
Note that the filename extension must be specified. If this is not done the compiler will return an error.
If the compilation process has finished correctly, we will find a file called Main.class in the same directory. This file contains the code of our compiled program (bytecode) and will be used in its execution.
To run the program we use the java command specifying the name of the class that contains the main() method, as shown below:
java Main
Once executed, we will see that “Hello, World!” is printed in the terminal:
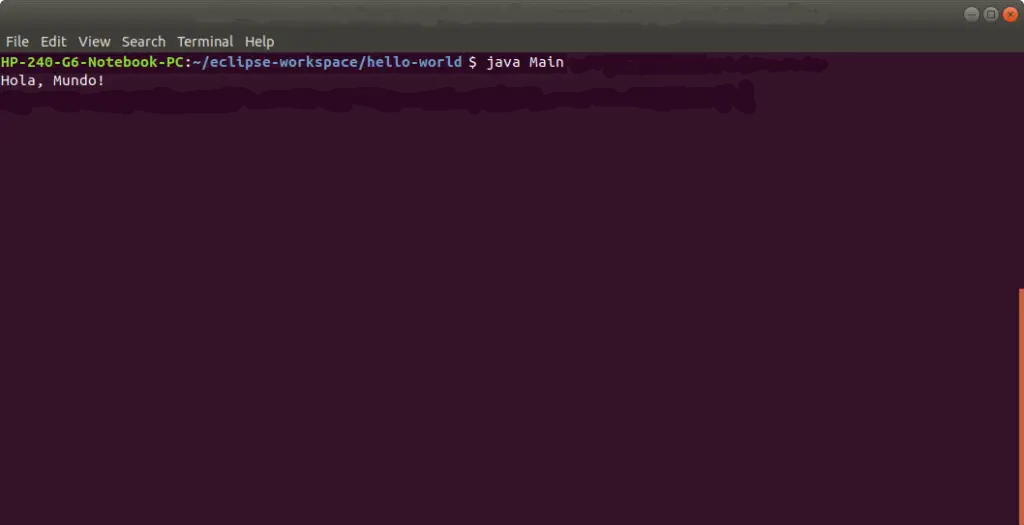
Conclusion
In this article we have seen how to create a first program in Java, which serves as a simple but essential introduction to programming, laying the foundation for more complex projects in the future. As you continue on your learning path, remember that practice, patience and the will to learn are key to mastering this versatile and powerful language.